Go string handling overview cheat sheet 40+ essential string functions: literals, concatenation, equality, ordering, indexing, UTF-8, search, join, replace, split, trim, strip, lowercase/uppercase. Go Cheat Sheet Credits Most example code taken from A Tour of Go, which is an excellent introduction to Go. Golang cheat sheet programming language reference card. Golang Cheat Sheet by deleted - Cheatography.com Created Date: 3158Z. Golang Cheatsheet As I’m learning a new language, it’s a very useful exercise for me to summarize the essence of the language for future personal reference. Golang is an excellent language to learn, and instead of having to Google and juggling between Go’s Playground, StackOverflow, Golang’s Documentation, it’s a lot nicer to have my.

It’s a reverse dictionary of printing format of fmt package. It let you find the right verbs from desired output quickly.
the value in a default format | %v |
type of the value | %T |
+255 | Always show sign | %+d |
‘ÿ’ | Single quoted | %q |
377 | Octal without leading '0’ | %o |
0377 | Octal with leading '0’ | %#o |
ff | Hex lowercase | %x |
FF | Hex uppercase | %X |
0xff | Hex with “0x” | %#x |
U+00FF | Unicode | %U |
U+00FF 'ÿ’ | Unicode with char | %#U |
11111111 | Binary | %b |
| 1| | 12| | 123| | 1234| |12345| |123456| |1234567| | |1 | |12 | |123 | |1234 | |12345| |123456| |1234567| | Minimum width=5. Expand if wider. | %5d %-5d (Left justify) |
| 1| | 12| | 123| | 1234| |12345| |12345| |12345| | |1 | |12 | |123 | |1234 | |12345| |12345| |12345| | Width=5. Truncate right if wider. | fmt.Printf(“%s”, fmt.Sprintf(“%5d”, value)[:5])) fmt.Printf(“%s”, fmt.Sprintf(“%-5d”, value)[:5])) (Left justify) or fmt.Printf(“|%5.5s|n”, strconv.Itoa(value)) fmt.Printf(“|%-5.5s|n”, strconv.Itoa(value)) (Left justify) |
| 1| | 12| | 123| | 1234| |12345| |23456| |34567| | |1 | |12 | |123 | |1234 | |12345| |23456| |34567| | Width=5. Truncate left if wider. | fmt.Printf(“%5d”, val % 100000) fmt.Printf(“%-5d”, val % 100000) (Left justify) |
|0012345| | Pad with '0’ (Right justify only) | %07d |
original | %f (=%.6f) 6 digits after decimal | %e (=%.6e) 6 digits after decimal | %g smallest number of digits necessary |
---|
Data Types
Constant
value never changes, can be number, bool, or string
const s string = 'banana'
get type of data structure
import reflect
myVar := 'blah'
fmt.Println(reflect.TypeOf(myVar).String())
int8 (-128 to 127)
int16 (-32,768 to 32,767)
int32 (2,147,483,648 to 2,147,483,647)
int64 (-9,223,372,036,854,775,808 to 9,223,372,036,854,775,807)
uint8, 16, 32, 64
float 32, 64
Structs (similar to Class)
type person struct {
name string
age int
}
func main() {
fmt.Println(person{'bob', 20}) ## {bob, 20}
s := person{'joe',35}
fmt.Println(s.name) ## joe
}
Array
var a [5]int
b := [5]int{1, 2, 3, 4, 5}
fmt.Println('array is:', b)
double arrayfmt.Println('array is:', b)
Hashes / Dictionary (Map)
package main
import 'fmt'
func main() {
prices := make(map[string]float64)
prices['OCKOPROG'] = 89.99
prices['ALBOÖMME'] = 129.99
prices['TRAALLÅ'] = 49.99
for key, value := range prices {
fmt.Printf('Key: %stValue: %vn', key, value)
}
}
Slice (Dynamic Array)
primes := [6]int{2,3,5,7,11,13} #array of prime numbers
var s []int = primes[1:4] # slice of prime array, values are from 1 to 4 index
var s []int = primes[:4] # slice, values from 0 to 4 index
len(s) # length of slice
cap(s) # max length of slice
Map - like a python Dictionary
JSON
read in a struct, generate a pretty print JSONGolang Cheat Sheet Pdf


Conditionals
For Loop
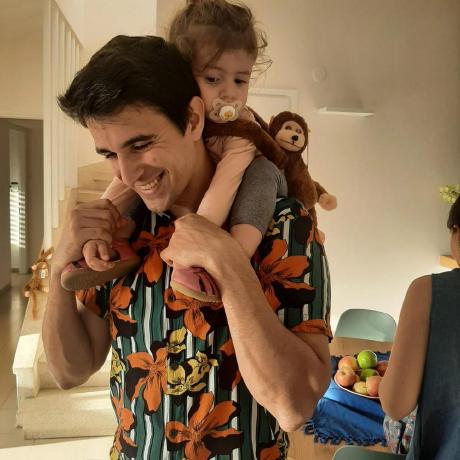
Switch Case
Functions
}## 1+2 = 3
multiple-value return function
func SumAndProduct(A, B int) (added, multiplied int) {
added = A+B
multiplied = A*B
return added, multiplied
}
Goto
func myFunc() {
i := 0
Here: // label ends with ':'
fmt.Println(i)
i++
goto Here //jump to label 'Here'
}
Parameters
package main
import (
'flag'
'fmt'
)
var name = flag.String('name', 'world', 'some name')
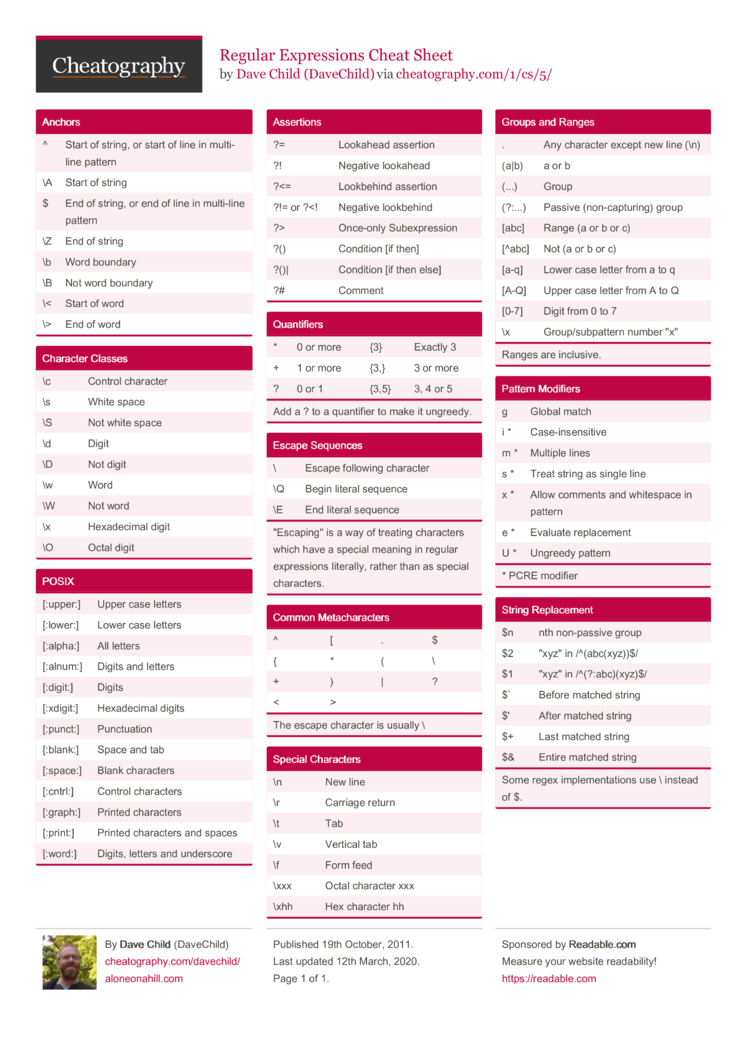
// var name, default value, description of param
func main() {
flag.Parse() //turn params into vars
fmt.Printf('hello %s', *name)
}
Rust Syntax Cheat Sheet
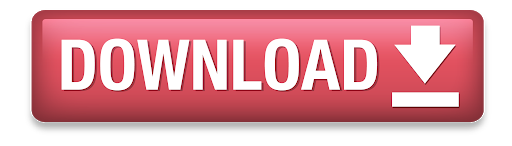